python 標準ライブラリのTkinterを使用して、デスクトップアプリを作ってみたいと思います
ファイルを実行すればウインドウが開き、アプリケーションとして様々な機能を持たせることができます
第3回としてEntry(エントリー)ウィジェットを使用して電卓っぽくしていきます
第2回の記事はこちら
・環境
windows 11
python 3.12.2
・Entry(エントリー)ウィジェットの生成
まず第1回で使用したソースコードを利用します
少しボタンを追加して電卓っぽくしていきます
import tkinter as tk
root = tk.Tk() # tkオブジェクトを、rootに格納
root.title("sample_App") # アプリのタイトルを決定
#root.geometry("600x400") # アプリ画面の大きさを設定
buttons = [
('7', 1, 0), ('8', 1, 1), ('9', 1, 2), ('/', 1, 3),
('4', 2, 0), ('5', 2, 1), ('6', 2, 2), ('*', 2, 3),
('1', 3, 0), ('2', 3, 1), ('3', 3, 2), ('-', 3, 3),
('0', 4, 0), ('.', 4, 1), ('=', 4, 2), ('+', 4, 3),
('C', 5, 0)
]
# ボタンの情報をリストで格納 (text, row, column)
for (text, row, col) in buttons:
button = tk.Button(root, text=text, font=("Arial", 20), padx=30, pady=30,)
# 親ウィジェット=root ボタンに表示するテキスト=text フォント="Arial",20 X方向の内部間隔=30 Y方向の内部間隔=30
button.grid(row=row, column=col)
# ボタングリッド指定
root.mainloop() #メインループ実行、アプリスタート
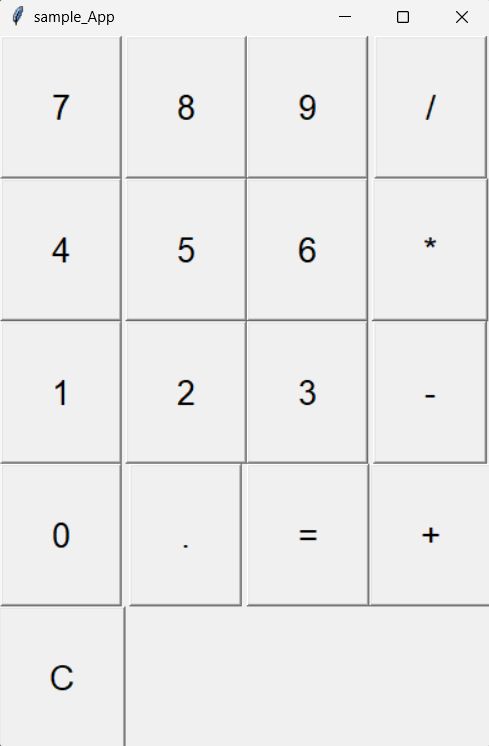
これにEntry(エントリー)ウィジェットを追加していきます
import tkinter as tk
def button_click(btm): # ボタンが押されたとき
curr =display_num.get() #現在の表示を取得、currに代入
display_num.set(curr + btm) #currと押したボタンを合わせて表示
root = tk.Tk()
root.title("calculator") # タイトル入力
display_num = tk.StringVar() # ウィジェット変数 文字列型 生成
display = tk.Entry(root, textvariable=display_num, font=("Arial", 18), width=14, justify="right")
# Entryウィジェットの生成 親ウィジェット=root テキスト変数display_num フォント=Arial サイズ=18
# 横幅(半角の文字数)=14 テキストの位置=右寄せ
display.grid(row=0, column=0, columnspan=4) #1行目 1列目で4つの列にまたがって配置
buttons = [
('7', 1, 0), ('8', 1, 1), ('9', 1, 2), ('/', 1, 3),
('4', 2, 0), ('5', 2, 1), ('6', 2, 2), ('*', 2, 3),
('1', 3, 0), ('2', 3, 1), ('3', 3, 2), ('-', 3, 3),
('0', 4, 0), ('.', 4, 1), ('=', 4, 2), ('+', 4, 3),
('C', 5, 0)
]
for (text, row, col) in buttons:
button = tk.Button(root, text=text, font=("Arial", 18), padx=20, pady=20,
command=lambda btm=text: button_click(btm))
# 親ウィジェット=root ボタンに表示するテキスト=text フォント="Arial", 18 X方向の内部間隔=20 Y方向の内部間隔=20
# クリック時の処理=ラムダ関数 引数btm=textでbutton_click(btm)実行
button.grid(row=row, column=col)
# ボタングリッド指定
root.mainloop()
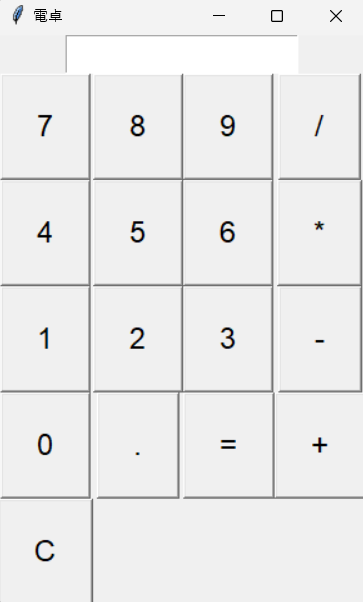
エントリーウィジェットが入って電卓らしくなりました
難しくなってきたのでコードの解説をします
display_num = tk.StringVar() で、ウィジェット変数の文字列型を生成します
display = tk.Entry(root, textvariable=display_num) でdisplayというエントリーウィジェットを生成し、それにに先ほどの変数を表示させます
display.grid(row=0, column=0, columnspan=4) でグリッド指定で配置します
columnspan=4 は4列にわたって長く配置するというオプションです
ボタンがクリックされたときの処理を解説します
def button_click(btm)
curr =display_num.get() で現在のdisplay_numの値を取得しcurrに代入します
display_num.set(curr + btm) でdisplay_numにcurrと押したボタンを合わせて表示させます
ボタンのcommandで、関数lambda で引数btm=textで、関数button_click(btm)を呼び出します
これでエントリーウィジェットに押したボタンが表示されていくというところまでできました
次回で表示された文字を計算する処理を書いていきます
コメント