Discordは、音声・ビデオ通話、テキストチャットができるコミュニケーションツールです
もともとはゲーマー向けに作られましたが、現在では企業やコミュニティ、友人同士のやり取りにも広く使われています
主な特徴
- サーバー: ユーザーが自由に作成できるチャットルームのようなもの
- チャンネル: サーバー内でテキストや音声ごとに分けられるトークルーム
- ボイスチャット: リアルタイムで音声通話が可能
- ボット(Bot): 自動返信や機能追加ができるプログラム(例: 音楽再生、株価取得など)
- ロールと権限: ユーザーごとに権限を設定できる(管理者、メンバーなど)
今回はDiscordのサーバーにbotを追加してメッセージを送信すると、返事を返すようにするシンプルな機能のbotをPythonを使って作成したいと思います
1.Discordにアカウント作成、ログイン
公式サイトの右上のログインからDiscordのアカウント作成またはログインをします

Discord - Group Chat That’s All Fun & Games
Discord is great for playing games and chilling with friends, or even building a worldwide community. Customize your own...
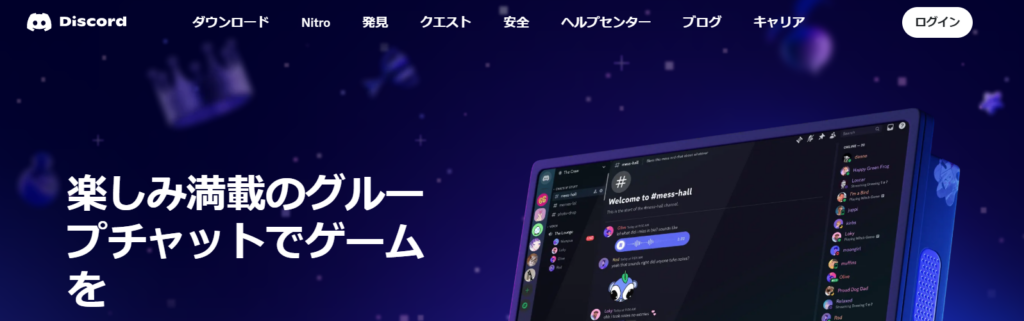
サーバーを作成
左側の + アイコンのサーバーを追加から、botを参加させるサーバーを作成します
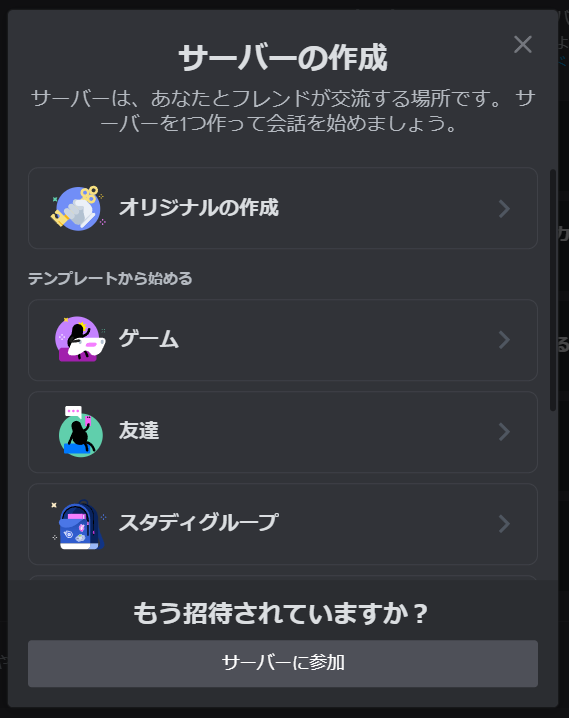
オリジナルの作成を選択、名前などを入力して新規作成を選択するとサーバーを作成できます
2.Discord Developer Portalでアプリを作成
Discord developer potalにアクセスします
Discord Developer Portal — API Docs for Bots and Developers
Integrate your service with Discord — whether it's a bot or a game or whatever your wildest imagination can come up with...

- 右上の「New Application」をクリックし、名前を設定します
- 左側のメニューから「Bot」を選択し、「Add Bot」をクリックします
- アイコンやバナーを選択して画像をアップロードします
Privileged Gateway Intentsの中の項目のPresence IntentとMessage Content Intentを有効にします
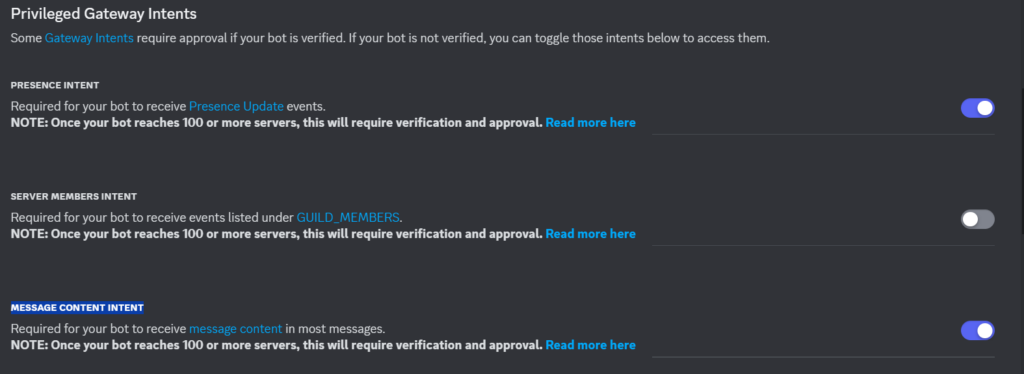
- Botの「TOKEN」をコピーしておきます

Botをサーバーに追加します
- 左メニューの「OAuth2」→「URL Generator」を開きます
- 「SCOPES」セクションで「bot」にチェックをいれます
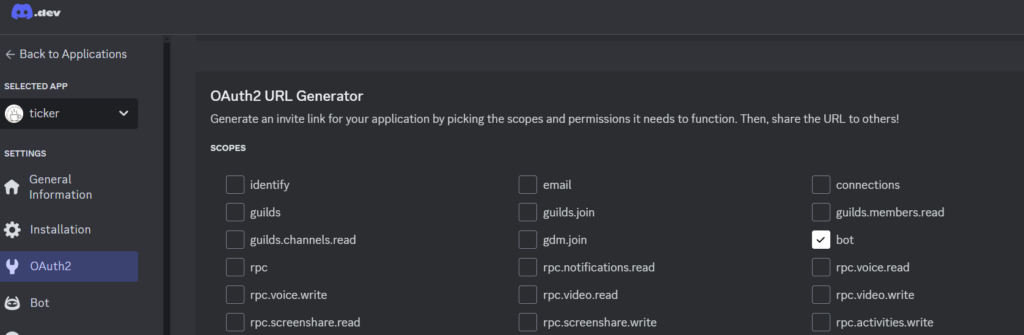
- 「BOT PERMISSIONS」で必要な権限を選択します
今回はメッセージや添付ファイルのやり取りをするので以下のPERMISSIONSにチェックをいれます
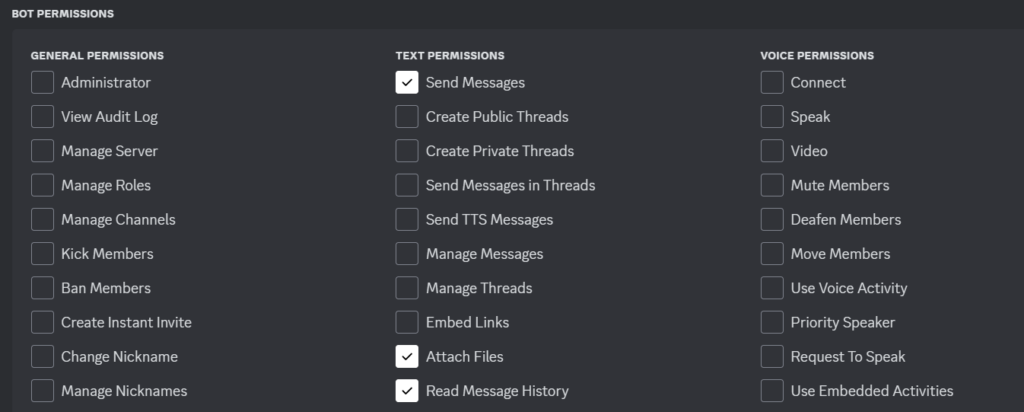
- 生成されたURLを新しくタブで開く
- botを先ほど作成したサーバーに招待する
- Discordのサーバーの画面に戻り、サーバーのテキストチャンネルに以下のようなメッセージが出てきたら、サーバーにbotが招待することができました

3.PythonでBotを作成
環境
- windows 11
- python 3.12.2
- discord.py 2.4.0
- python-dotenv 1.0.1
必要なライブラリをインストールします
pip install discord.py python-dotenv
botを動かすpythonファイルを作成します
import discord
from discord.ext import commands
import os
from dotenv import load_dotenv
# .envファイルを読み込む
load_dotenv()
# Discordのbotトークン
DISCORD_TOKEN = os.getenv("DISCORD_TOKEN")
TOKEN = DISCORD_TOKEN
intents = discord.Intents.default()
intents.message_content = True
bot = commands.Bot(command_prefix="!", intents=intents)
@bot.event
async def on_ready():
print(f"Logged in as {bot.user}")
@bot.command()
async def hello(ctx):
await ctx.send("Hello! I'm a bot!")
bot.run(TOKEN)
作業ディレクトリに.envファイルを作成します
DISCORD_TOKEN="XXXXXXXXXXXXXXXXX"
.envファイルの使い方は以前の記事で紹介しているので参考にしてください
4.コードの解説
import discord
from discord.ext import commands
- discord モジュールと、その中の commands をインポート
- discord はDiscord APIとやり取りするためのライブラリ
- commands はBotのコマンド機能を簡単に作るための拡張機能(discord.ext.commands)
Intents(意図)設定
intents = discord.Intents.default()
intents.message_content = True
- Intents はBotがどんな種類のイベントを受け取るかを設定する
- default() でデフォルトの設定を取得
- intents.message_content = True にすると、Botはメッセージ関連のイベントを受け取れるようになる
Botオブジェクトの作成
bot = commands.Bot(command_prefix="!", intents=intents)
- command_prefix=”!” :
!
をつけたメッセージをコマンドとして認識- !hello と入力すると hello コマンドが実行される
- intents=intents → 先ほど設定した意図を適用
Botがオンラインになった時の処理
@bot.event
async def on_ready():
print(f"Logged in as {bot.user}")
- @bot.event → イベント(特定のタイミングで実行される処理)を定義
- on_ready() → Botがオンラインになったら実行される関数
- print(f”Logged in as {bot.user}”) で、Botの名前をターミナルに表示
コマンドの作成
@bot.command()
async def hello(ctx):
await ctx.send("Hello! I'm a bot!")
- @bot.command() → コマンドを作成するデコレーター(関数の前につけて機能を追加)
- async def hello(ctx): → !hello と入力されたら実行される関数
- await ctx.send(“Hello! I’m a bot!”) → メッセージを送信
botの実行
bot.run(TOKEN)
- bot.run(TOKEN) でBotを実行し、Discordに接続
botの起動
ターミナルでbotを動かすpythonファイルを実行するとbotが起動します
起動したらDiscordのサーバーの画面からtickerの部分をクリックしてbotにメッセージを送信します

このbotは !hello と入力すると Hello!I’m a bot! と答えるようになっています

このようにbotがちゃんと反応してくれました
今後の展開
このbotのコマンドを他にも増やして、Web APIとも連携して、簡単に株価情報を取得する機能を追加したいと思います
コメント